Design Elaboration
Elaboration is the process that occurs after the code is parsed. From one or more starting points, called tops, all instances have their designs computed, parameters and other constants get their values, generate constructs are instantiated and port connections are established.
In the project build config file, specify the elaboration top(s) with one or more -top top_name directives.
E.g.
work.elem_name - from the specified library, the SystemVerilog *module*, SystemVerilog *configuration*, VHDL *entity* or VHDL *configuration* is set as the top
elem_name - all libraries are searched for the design element and the first found is set as top
elem_name:config - the configuration specified is the top, even in the case of a name collision with either a SystemVerilog module or a VHDL entity
If no directive is used, then the elaboration is preceded by a top candidates search through all the designs. A UNSPECIFIED_TOP warning is also displayed in the Console View to mark the absence of any chosen top design.
Note
SystemVerilog modules can be instantiated by a VHDL entity and vice versa. You can also specify design tops from either language.
Top candidates
When no top design element is specified in the project build config file, then any design element that satisfies the following requirements is considered a top:
The design element is either a SystemVerilog module, a SystemVerilog program, a VHDL entity or a VHDL configuration. Do note that a SystemVerilog configuration cannot be a candidate.
The design element is not instantiated anywhere. Even instantiations under inactive generate constructs are considered and rule out the element in question.
Customizable: The design element has sub-instances or local generate constructs. This requirement can be disabled by specifying in the project build config the following directive:
+dvt_enable_elaboration_empty_tops+true
.
As mentioned before, a mixed-language project can have any combination of SystemVerilog, SystemVerilog or VHDL top candidates.
Parameter values
During elaboration, parameters (or generics in VHDL) get their values computed. The following sources, ordered by importance, are searched:
Configuration overrides. Any override of a parameter in a SystemVerilog config or a VHDL configuration rule is considered first.
configuration cfg of top_entity
for rtl
for inst : aent use entity work.aent
generic map (PARAM => 1);
end for;
end for;
end configuration;
Defparam assignments
Instantiation overrides
Default values
The computed value can be seen in the parameter tooltip.
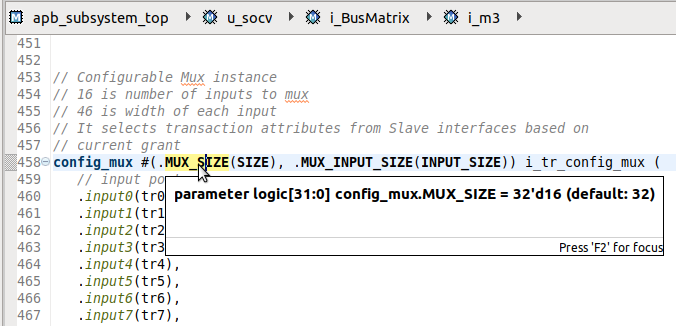
The Inspect View will also show it, alongside number base conversions.
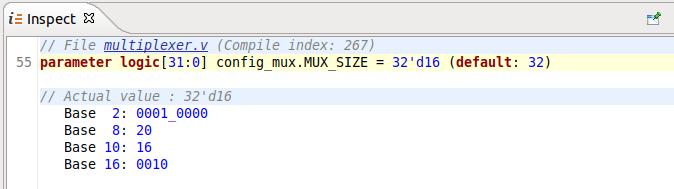
To easily jump to the driver or source of the parameter value, you can use the Jump To Assignment hyperlink.
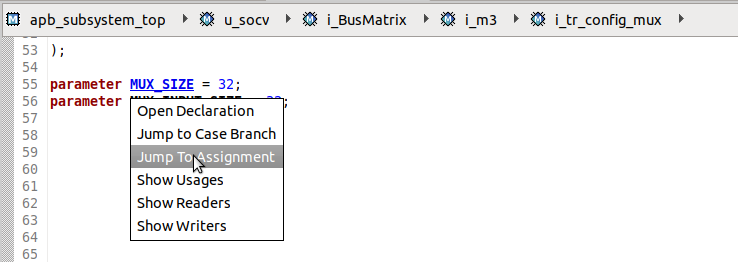
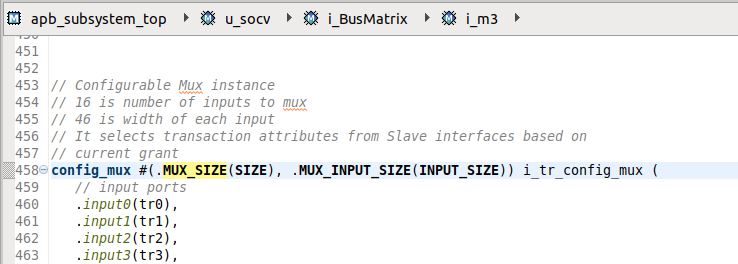
Note
The current design path, shown in the Design Breadcrumb, is used for determining the instance to which the inspected parameter belongs.
Unelaborated Design
The unelaborated design is the part of the compiled code not found under any of the elaboration tops. It can divided into two categories:
Inactive generate constructs: e.g. the else branch of an if generate, all inactive case items, for loop generates with no iterations
Design elements not instantiated under the elaboration tops: unused interfaces, the design of an instance under an inactive generate construct
Unelaborated modules/programs/interfaces/checkers/entities are marked with warnings. Also, packages that are not used in the elaborated design are marked with UNELABORATED_PACKAGE warnings.
Tool functionality in the unelaborated part of the design can be restricted through the +dvt_unelaborated_compile_checks All Build Directives. A faster build time is the main benefit. But, the tradeoff is the loss of all IDE specific functionality, like for example Show Usages, Readers or Writers, Rename Refactoring, or Design Diagrams, in the excluded code.
Debugging
Debugging the elaboration is possible through the +dvt_elaboration_debug
global build config directive. Its output is shown in the project DVT Console/build log. It accepts multiple argument values:
E.g. +dvt_elaboration_debug+STATS+EVAL
STATS |
enables various statistics, e.g. number of elaborated instances, number of defparams found |
MEMORY |
the elaborated hierarchy, both designs and parameter values, is listed |
EVAL |
internal unsuccessful evaluations are marked with an UNKNOWN_EXPRESSION error that appears also in the Problems View |
INCREMENTAL |
additional information regarding incremental adaptive elaboration is shown |
BINDS_PASS |
additional information regarding the elaboration of bind directives is shown |
SUBINSTANCE_COPIES |
additional information regarding the SINGLE_PASS elaboration of sub-instances that get copied |
Performance
The following build config directives can restrict the elaboration and therefore improve performance when needed.
|
Disable or change steps in the elaboration.Use EACH_GENERATE_BLOCK_ONCE to elaborate each generate block, whether active or inactive, only once.
All branches of conditional generate blocks will be elaborated. Loop generate blocks will be elaborated only once, for index 0.
Similarly, an array of instances will have only one element per dimension, corresponding to index 0.Use NO_GENERATE_BLOCKS to not elaborate generate blocks.
Any sub-instance found under a generate block will also not be elaborated.Use NO_PARAM_EVAL to disable parameter evaluation. Parameters and constants will not have their values computed, not even their default values.
Use EACH_SPEC_ONCE to stop the SINGLE_PASS elaboration of an instance whose design specialization has already been elaborated (the instance is BBOX-ed). Use NO_SUBINSTANCE_COPIES to not optimize the SINGLE_PASS elaboration through copies of identical sub-instances.
|
E.g. |
|
Configure the cut-off number for elaborated loop blocks.In a design with many loop generate constructs, limiting the number to 1 per loop can drastically reduce the elaboration time. |
E.g. |
|
Configure the cut-off number for elaborated function loop statements.In a design with many evaluated functions, limiting the number to 100 per loop statement (e.g. for, foreach, while) can drastically reduce the elaboration time. Functions affected by the cutoff are not elaborated. |
E.g. |
|
Configure the kind of elaboration performed at full build.Use SINGLE_PASS to have the elaboration be performed in one pass.
A notable performance improvement can be observed but note that any usage of defparam assignments or configurations may lead to unexpected elaboration results.Use MULTI_PASS to have the elaboration use multiple passes. In most cases it is slower than the SINGLE_PASS elaboration, but ensures correct handling of defparam assignments and configurations.Use ADAPTIVE to let the tool determine the kind based on multiple criteria.
|
E.g. |
|
Configure the maximum number of passes allowed when resolving bind directives. |
E.g. |
|
Disable function call evaluation in elaboration. |
E.g. |
|
Skip defparam assignments during elaboration. |
E.g. |
|
Skip elaboration of an instance based on design name.Instance port connections and parameter overrides are not checked and sub-instances are not elaborated.
Skipped designs are considered unelaborated.Elaboration tops cannot be skipped.Wildcards such as ‘*’ (any string) and ‘?’ (any character) can be used in the pattern.
|
E.g. |
|
Skip elaboration of an instance based on design file absolute path.Instance port connections and parameter overrides are not checked and sub-instances are not elaborated.
Skipped designs are considered unelaborated.Elaboration tops cannot be skipped.Wildcards such as ‘*’ (any string) and ‘?’ (any character) can be used in the pattern.
|
E.g. |
|
Skip elaboration of an instance based on hierarchical path.Instance port connections and parameter overrides are not checked and sub-instances are not elaborated.
Skipped designs are considered unelaborated.Elaboration tops cannot be skipped.Wildcards such as ‘*’ (any string) and ‘?’ (any character) can be used in the pattern.
|
E.g. |
|
Black box an instance based on design name.Instance port connections and parameter overrides are checked but sub-instances are not elaborated.
Black boxed designs are considered unelaborated. In the Design Hierarchy View, they are marked as BLOCK BOX.Elaboration tops cannot be black boxed.Wildcards such as ‘*’ (any string) and ‘?’ (any character) can be used in the pattern.
|
E.g. |
|
Black box an instance based on design file absolute path.Instance port connections and parameter overrides are checked but sub-instances are not elaborated.Black boxed designs are considered unelaborated.
In the Design Hierarchy View, they are marked as BLOCK BOX.Elaboration tops cannot be black boxed.Wildcards such as ‘*’ (any string) and ‘?’ (any character) can be used in the pattern.
|
E.g. |
|
Black box an instance based on hierarchical path.Instance port connections and parameter overrides are checked but sub-instances are not elaborated.Black boxed designs are considered unelaborated.
In the Design Hierarchy View, they are marked as BLOCK BOX.Elaboration tops cannot be black boxed.Wildcards such as ‘*’ (any string) and ‘?’ (any character) can be used in the pattern.
|
E.g. |
The following build config directives can restrict the functionality in the unelaborated code in order to improve performance.
|
Control the scope of the unelaborated design build checks and functionality.
If set to
NONE , functionality and checking in the unelaborated modules and unelaborated local generate blocks is limited.If set to
GENERATE_BLOCKS , functionality and checking in all unelaborated modules is limited.If set to
DISCRETE , functionality and checking in the unelaborated modules found in library files is limited. This is the default value of the directive.If set to FULL, functionality and checking is not limited in the unelaborated code. |
E.g. |
|
Disable unelaborated package constants evaluation and checking. |
E.g. |